この記事は最終更新日から1年以上が経過しています。
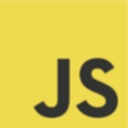
投稿日 2019/12/17
更新日 2019/12/17 ✏
CSSで簡単なアニメーション
HTMLのボタン要素の動きや変化などのちょっとしたアニメーションをCSSで実現する方法です。
今回紹介するサンプルコードでは、animeffect()
というオリジナルな汎用ヘルパー関数を定義して利用していますが、この関数はコピペでそのまま使えます。(要jQuery)
目次
実装手順
基本的な実装手順は以下のようになります。以下は、クリックされたボタン要素を3回フラッシュさせるアニメーションの例です:
- スタイルシートに
@keyframe
(アニメーションの動き)とそれを利用するクラスを実装:
@keyframes flash {
0% {
opacity: 1;
}
50% {
opacity: 0.5;
}
100% {
opacity: 0;
}
}
.flash {
/* 適用keyframe名: */
animation-name: flash;
/* 1回のアニメーション周期が完了するまでの所要時間: */
animation-duration: 0.3s;
/* アニメーションのタイミング・進行割合: */
animation-timing-function: linear;
/* 繰り返し回数: */
animation-iteration-count: 3;
/* 開始タイミング: */
animation-delay: 0s;
}
- アニメーションを適用するヘルパー関数
animeffect(event, '<cssクラス名>', <適用ミリ秒>)
をグローバルに定義:
/**
* アニメーションを適用するヘルパー関数
* @param {Event} e
* @param {String} cls - cssクラス名
* @param {Number} msec - [OPTIONAL] クラスを戻す経過時間。デフォルトは1000ミリ秒
*/
function animeffect(e, cls, msec = 1000) {
const $self = $(e.currentTarget);
$self.addClass(cls);
setTimeout(() => {
$self.removeClass(cls);
}, msec);
}
NOTE:こちらの関数は jQuery を利用しています。
- アニメーションを適用したい要素のイベントハンドラで
animeffect()
をコール: (ここではボタン要素のonclick
属性に直接記述しました)
<button onclick="animeffect(event, 'flash')">click to flash</button>
これで、クリックすると3回フラッシュするアニメーションがボタン要素に適用されました。
サンプルコード
以下のサンプルコードでは、先ほど紹介した「フラッシュ」の他にも、「回転」や「幅スケール」、「スライド」といったアニメーションも追加しています。
<!DOCTYPE html>
<html>
<head>
<title>CSS Animation</title>
<script src="https://code.jquery.com/jquery-3.4.1.min.js" integrity="sha256-CSXorXvZcTkaix6Yvo6HppcZGetbYMGWSFlBw8HfCJo=" crossorigin="anonymous"></script>
<style type="text/css">
/******************************************************/
/* フラッシュ (不透明度100% -> 50% -> 0%)
/******************************************************/
@keyframes flash {
0% {
opacity: 1;
}
50% {
opacity: 0.5;
}
100% {
opacity: 0;
}
}
.flash {
animation-name: flash;
animation-duration: 0.3s;
animation-timing-function: linear;
/* 3回繰り返し: */
animation-iteration-count: 3;
/*animation-delay: 2s;*/
}
/******************************************************/
/* 回転 (360度回転)
/******************************************************/
@keyframes spin {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
}
.spin {
animation-name: spin;
animation-duration: 0.3s;
animation-timing-function: linear;
animation-iteration-count: 1;
/*animation-delay: 2s;*/
}
/******************************************************/
/* 幅のスケール (幅120px -> 180px -> 340px -> 120px)
/******************************************************/
@keyframes scale {
0% {
width: 180px;
}
50% {
width: 340px;
}
100% {
width: 120px;
}
}
.scale {
animation-name: scale;
animation-duration: 0.3s;
animation-timing-function: linear;
/* 3回繰り返し: */
animation-iteration-count: 3;
/*animation-delay: 2s;*/
width: 120px;
}
/******************************************************/
/* スライド (左から右へ)
/******************************************************/
@keyframes slide {
0% {
left: 0px;
}
50% {
left: 50%;
}
100% {
left: 100%;
}
}
.slide {
animation-name: slide;
animation-duration: 0.3s;
animation-timing-function: linear;
animation-iteration-count: 1;
/*animation-delay: 2s;*/
position: relative;
}
</style>
</head>
<body>
<div>
<button onclick="animeffect(event, 'flash')">click to flash</button>
<button onclick="animeffect(event, 'spin')">click to spin</button>
<button onclick="animeffect(event, 'scale')">click to scale</button>
<div style="background: gray; width: 300px;">
<button onclick="animeffect(event, 'slide', 300)">click to slide</button>
</div>
</div>
<script type="text/javascript">
/**
* アニメーションを適用するヘルパー関数
* @param {Event} e
* @param {String} cls - cssクラス名
* @param {Number} msec - [OPTIONAL] クラスを戻す経過時間。デフォルトは1000ミリ秒
*/
function animeffect(e, cls, msec = 1000) {
const $self = $(e.currentTarget);
$self.addClass(cls);
setTimeout(() => {
$self.removeClass(cls);
}, msec);
}
</script>
</body>
</html>
サンプルコードを実行
サンプルコードを実行するとこんな感じになります:
以上となります。